ASP.NET MVC Pattern
A design pattern for achieving a clean separation of concerns
Supported on Windows, Linux, and macOS
Model View Controller (MVC)
MVC is a design pattern used to decouple user-interface (view), data (model), and application logic (controller). This pattern helps to achieve separation of concerns.
Using the MVC pattern for websites, requests are routed to a Controller that is responsible for working with the Model to perform actions and/or retrieve data. The Controller chooses the View to display and provides it with the Model. The View renders the final page, based on the data in the Model.
MVC with ASP.NET
ASP.NET gives you a powerful, patterns-based way to build dynamic websites using the MVC pattern that enables a clean separation of concerns.
public class Person
{
public int PersonId { get; set; }
[Required]
[MinLength(2)]
public string Name { get; set; }
[Phone]
public string PhoneNumber { get; set; }
[EmailAddress]
public string Email { get; set; }
}
Models & data
Create clean model classes and easily bind them to your database. Declaratively define validation rules, using C# attributes, which are applied on the client and server.
ASP.NET supports many database engines including SQLite, SQL Server, MySQL, PostgreSQL, DB2 and more, as well as non-relational stores such as MongoDB, Redis, and Azure Cosmos DB.
public class PeopleController : Controller
{
private readonly AddressBookContext _context;
public PeopleController(AddressBookContext context)
{
_context = context;
}
// GET: /people
public async Task Index()
{
return View(await _context.People.ToListAsync());
}
// GET: /people/details/5
public async Task Details(int id)
{
var person = await _context.People.Find(id);
if (person == null)
{
return NotFound();
}
return View(person);
}
}
Controllers
Simply route requests to controller actions, implemented as normal C# methods. Data from the request path, query string, and request body are automatically bound to method parameters.
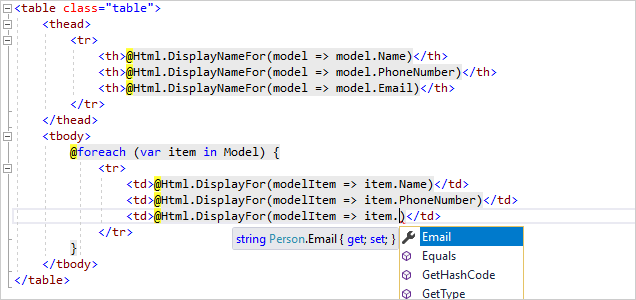
Views with Razor
The Razor syntax provides a simple, clean, and lightweight way to render HTML content based on your view. Razor lets you render a page using C#, producing fully HTML5 compliant web pages.
Ready to get started?
Our step-by-step tutorial will help you get MVC with ASP.NET running on your computer.